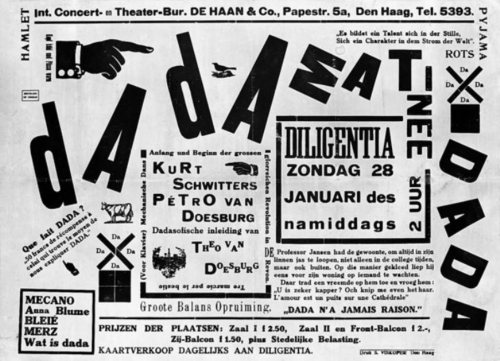
Introduction
In this article we will see how to extract the main text content from a blog using the Google Reader NoAPI.
Extracting the main text content from a web page is an important step in the text processing pipeline. The source code of pages in HTML is usually cluttered with advertising and other text which is not related to the main content. Formally, in the context of computer science, it is impossible for a computer to distinguish between the main content and other content on the same page. That is, no algorithm can recognize it for all possible cases. Sometimes it is even difficult for humans to distinguish it. Recognition of primary content is part of the machine learning/artificial intelligence field of study.
In practice there are many ways to recognize main content. If, for example, a blog platform includes attributes which indicate where the main content is, the process will be straightforward. Similarly, If the pages on a particular site have a well defined structure, we can also infer where the main content is by sampling a few pages. In this approach, we train the recognizer to apply patterns to additional pages. Of course purely manual work is another option. The quickest way to build an army of human recognizers is to put the job on sites like Amazon’s Mechanical Turk or similar services such as Microworkers.
For a good compilation of resources related to this subject you can see:
Extracting the Main Content from a Blog
If the blog platform includes information about the main text content on their tags, making an XPath expression for each one will do the trick. Now imagine that you want to do it automatically, without depending on each blog platform or blog theme. In this case you can read the RSS feed, which generally only includes main text, and extract the text from there. However, not all blogs post the complete text in the feed. The TechCrunch feed, for example, shows the first part of the text, but you have to click to continue reading. In this case you can use the partial text from the feed to recognize the complete text in the HTML. A potential problem with reading RSS feeds is that they only contain the most recent articles. To get around this limitation, we can get a longer feed history from Google Reader. Google Reader has some gaps and misses some articles, but this issue is beyond the scope of this article.
Getting Blog Text from Google Reader
Since Google Reader does not have a real API we will rely on the Google Reader API lib by Mauro Asprea from Wish and BAM!. He is an active reader of this blog and a friend.
We will retrieve posts by Fred Wilson, one of the most prolific VC bloggers, since he has blogged since 9/23/2003 on an almost daily basis, and includes the whole post within the feed.
Python code
#!/usr/bin/python
# *-* coding: utf-8 *-*
import sys
import time
from GoogleReader import CONST
from GoogleReader.reader import GoogleReader
import lxml.html
USERNAME = '' # Replace with your Google Reader username
PASSWORD = '' # Replace with your Google Reader password. Not included in this post :-)
gr = GoogleReader()
login_info = (USERNAME, PASSWORD)
gr.identify(*login_info)
gr.login()
gr.add_subscription(url="http://feeds.feedburner.com/avc")
xmlfeed = gr.get_feed(url="http://feeds.feedburner.com/avc")
COUNT = 1000
i=0
print >>sys.stderr, "page:", i
for entry in xmlfeed.get_entries():
print entry['title'].encode('utf-8'), time.ctime(entry['published'])
doc = lxml.html.fromstring(entry['content']) # Thanks lxml.html for handling incomplete HTML documents!
print doc.text_content().encode('utf-8')
print "******************************************************************************************************"
continuation = xmlfeed.get_continuation()
i+=1
while continuation != None and i < COUNT:
print >>sys.stderr, "page:", i
xmlfeed = gr.get_feed(url="http://feeds.feedburner.com/avc", continuation = continuation)
for entry in xmlfeed.get_entries():
print entry['title'].encode('utf-8'), time.ctime(entry['published'])
try:
doc = lxml.html.fromstring(entry['content']) # Thanks lxml.html for handling incomplete HTML documents!
print doc.text_content().encode('utf-8')
except:
print "------------------ ERROR -------------------"
print entry['content']
print "******************************************************************************************************"
continuation = xmlfeed.get_continuation()
i+=1
Notes
If you try this script you will realize that the oldest post retrieved is from 9/29/2005. The real first post however was on 9/23/2003. Why don’t we see it? I believe it is because Google Reader uses feed information from FeedBurner, which was launched in 2004 and acquired by Google in 2007, so they probably started recording feed entries then. Incidentally Union Square Ventures was one of the original FeedBurner investors.
There is an easier way to retrieve text in the specific case of Fred Wilson’s blog and other HTML5 modern sites. HTML5 provides an <article> tag, so you can just crawl the whole site and retrieve the content within the <article> tag. You’ll need an extra step to deduplicate the content since many of the crawled pages will appear more than once. For example if you follow categories like MBA Mondays you will find articles that also appear when you follow another path.
Lessons Learned
- We can use Google Reader to easily extract text content from blogs.
- Google Reader has its limitations: it doesn’t cover posts before a certain data and sometimes skips posts.
- HTML5 adds a valuable new tag for differentiating article text from the rest of the content.
See Also
- Voice Recognition + Content Extraction + TTS = Innovative Web Browsing
- Google Search NoAPI
Additional Resources
- Newspaper: News, full-text, and article metadata extraction in Python 3
- boilerpipe: Boilerplate Removal and Fulltext Extraction from HTML pages
- Readability API
- HTML Content Extraction Questions on StackOverflow
- Google Reader Development Questions on StackOverflow